ここでは、flutterのリストビュー(ListView) をドラッグアンドドロップする ReorderableListView を紹介します。Todoリストを表示している際に順番を入れ替えるようなことができますね。
ListViewでドラッグアンドドロップを行うサンプル
Todoリストのサンプルです。こんな感じに動作させることができるようになります。
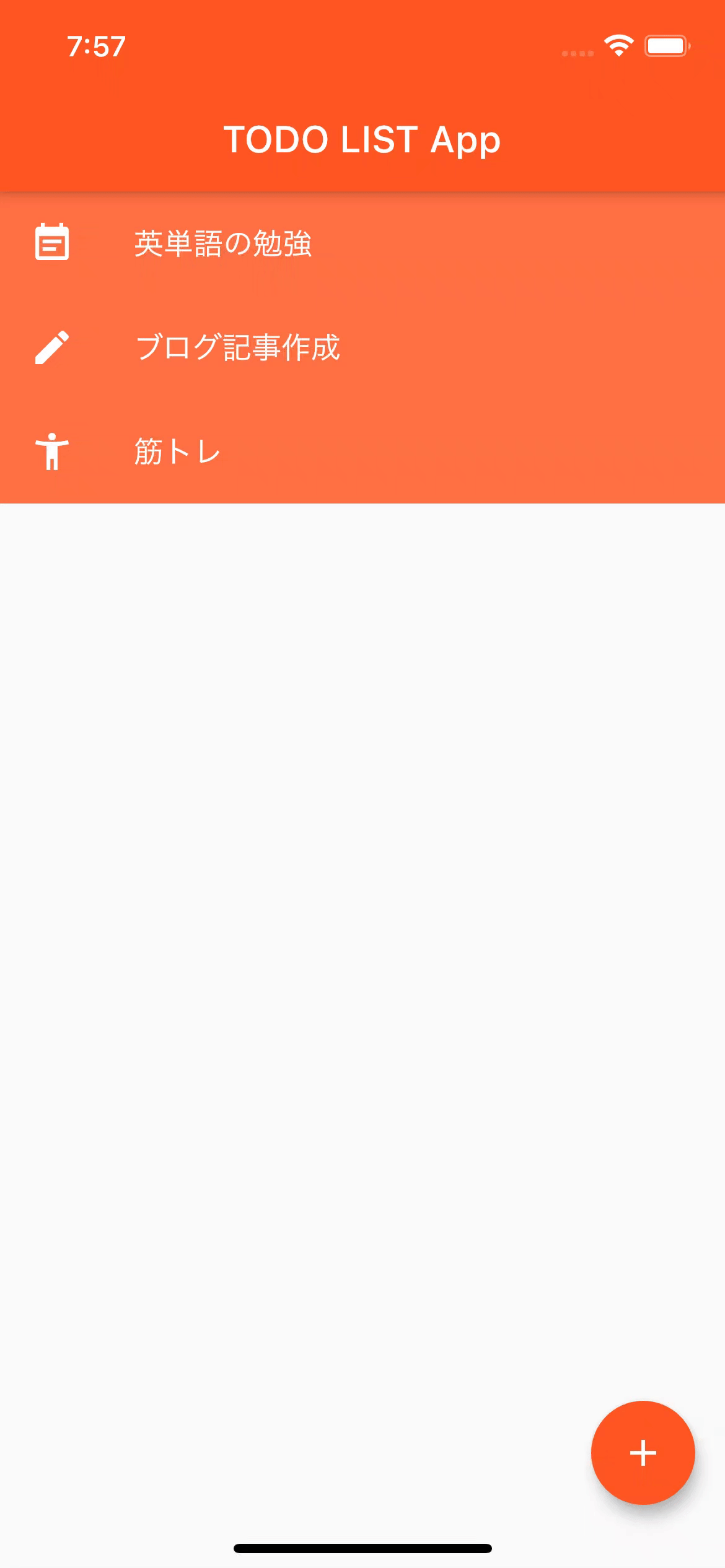
ReorderableListViewの使い方
ドラッグアンドドロップには、ReorderableListView を利用します。パッケージの追加は必要ありません。
リストの各項目を一意に識別するために、Keyプロパティを追加する必要があります。
ドラッグアンドドロップの際に、この onReorder が呼ばれます。公式のコードは stateful を使った例になっていますが、次のコードは状態遷移に riverpod を利用している例になります。
onReorder: (oldIndex, newIndex) {
todo.dragAndDrop(oldIndex, newIndex);
},
class Todo with ChangeNotifier {
void dragAndDrop(int oldIndex, int newIndex) {
if (oldIndex < newIndex) {
newIndex -= 1;
}
TodoModel todoModel = todoList.removeAt(oldIndex);
todoList.insert(newIndex, todoModel);
notifyListeners();
}
}
全体のコード
import 'package:flutter/material.dart';
import 'package:flutter_hooks/flutter_hooks.dart';
import 'package:hooks_riverpod/hooks_riverpod.dart';
final todoProvider = ChangeNotifierProvider((ref) => Todo());
void main() {
runApp(
ProviderScope(child: MyApp()),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.deepOrange,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage());
}
}
class MyHomePage extends HookWidget {
@override
Widget build(BuildContext context) {
final Todo todo = useProvider(todoProvider);
return Scaffold(
appBar: AppBar(
title: Text("TODO LIST App"),
),
body: Container(
child: ReorderableListView(
children: todoList.map((TodoModel todoModel) {
return Container(
key: Key(todoModel.getKey),
decoration: BoxDecoration(color: Colors.deepOrange[400]),
child: ListTile(
leading: Icon(
todoModel.icon,
color: Colors.white,
),
title: Text(
todoModel.getTitle,
style: TextStyle(color: Colors.white),
),
),
);
}).toList(),
onReorder: (oldIndex, newIndex) {
todo.dragAndDrop(oldIndex, newIndex);
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.add),
),
);
}
}
class Todo with ChangeNotifier {
void dragAndDrop(int oldIndex, int newIndex) {
if (oldIndex < newIndex) {
newIndex -= 1;
}
TodoModel todoModel = todoList.removeAt(oldIndex);
todoList.insert(newIndex, todoModel);
notifyListeners();
}
}
class TodoModel {
String title;
IconData icon;
String key;
TodoModel({this.title, this.icon, this.key});
String get getTitle => title;
IconData get getIcon => icon;
String get getKey => key;
}
List todoList = [
TodoModel(
title: "英単語の勉強",
icon: Icons.event_note,
key: "1",
),
TodoModel(
title: "ブログ記事作成",
icon: Icons.edit,
key: "2",
),
TodoModel(
title: "筋トレ",
icon: Icons.accessibility,
key: "3",
),
];
ITエンジニアが利用したい転職エージェントNo.1
まとめ
以上で ListView をドラッグアンドドロップできる ReorderableListView の使い方は終わりになります。かなり簡単に実装できるので、Todo リストの勉強にも良いと思います。
副業プログラミングで稼ぐやり方は、masamaru blogで解説していますのでぜひチェックしてみてください。
masamaru blog – 副業プログラミング、ブログ情報
転職を目指しているITエンジニアの方は、レバテックキャリアがおすすめですよ。